You’re definitely searching for a quick and easy approach to query and deal with data if you’re working with C# and frequently handle data from several sources, such as databases, XML files, or in-memory collections. Let me introduce you to C#’s sophisticated LINQ (Language Integrated Query) technology, which makes it easier to query and manipulate data right within your code.
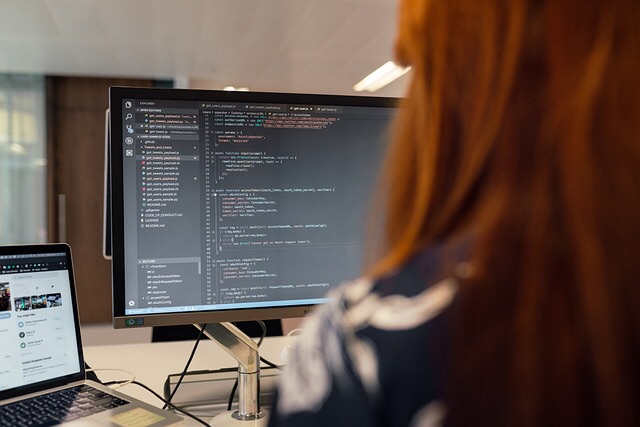
By making your code more legible and succinct, learning LINQ may greatly increase your productivity, regardless of your level of experience with C#. Companies should hire dedicated C# developers that can contribute their LINQ knowledge if you’re considering incorporating LINQ into your project but lack the time or know-how.
What is LINQ?
Language Integrated Query, or LINQ, is a collection of C# built-in methods that make it easier and more natural to run queries on data sets. Before LINQ, sophisticated loops, conditions, and human data manipulation were frequently required for data searching and filtering. This is changed by LINQ, which makes your code clearer and simpler to read by allowing you to query any data source using a standard syntax.
Why LINQ is a Game-Changer for C# Developers
LINQ’s primary benefit is that it enables you to construct SQL-like queries in C#. Because you don’t have to go through every item by hand to get or filter particular data, this may greatly simplify the code. Sorting, filtering, and grouping are just a few of the processes that may be combined in a single statement using LINQ queries in an easy-to-read manner.
1. Simplified Syntax
When working with data, one of the most difficult things for developers to deal with is the intricacy of creating loops and conditions. By offering a declarative syntax that is simple to comprehend and use, LINQ streamlines this procedure. With LINQ, you can describe data operations in a concise and legible manner rather than writing long code with several loops and conditionals.
2. Readable Code
By eliminating boilerplate code and offering a more expressive syntax, LINQ improves the readability of your work. The more complicated your projects get, the more crucial understandable code becomes. LINQ facilitates data operations by providing methods such as Where, Select, OrderBy, GroupBy, and several more. These techniques improve the readability and error-proneness of your code.
3. Less Error-Prone
Errors frequently occur while writing loops and manually filtering or sorting data, particularly when dealing with complicated situations. By abstracting routine processes into established methods, LINQ lowers the possibility of mistakes. Because LINQ optimizes and tests the underlying operations, you can concentrate on the higher-level logic of your code.
4. Integration with Multiple Data Sources
The flexibility of LINQ is among its finest qualities. Although LINQ began with in-memory collections, it may currently query a wide range of data sources, including:
- LINQ to Objects – To query in-memory collections that implement IEnumerable, such as lists, arrays, and other collections.
- LINQ to SQL – For running queries on relational databases such as SQL Server.
- LINQ to XML – For querying and manipulating XML data.
- LINQ to Entities – For working with the Entity Framework to query databases using LINQ queries.
5. Deferred Execution and Performance
A lot of the time, LINQ queries are lazy, meaning they wait until the data is really needed. This allows you to chain many operations on a dataset and removes the need for unnecessary calculations up front. Performance is the benefit here as LINQ just runs queries when required and doesn’t calculate intermediate results until specifically asked for.
How to Get Started with LINQ?
You must first make sure you understand some of the most often used LINQ operators before beginning to work with LINQ, including:
- Where(): Uses a criterion to filter data.
- Choose (): Data is projected into a new form or structure using this function.
- OrderBy(),ThenBy(): Data is arranged according to a defined criterion by these functions.
- GroupBy(): Sorts data according to a given key.
- FirstOrDefault(), SingleOrDefault(): Returns a default value in the event that no match is found, or retrieves a single element from a collection.
Final Words
With its ability to handle numerous data sources and carry out operations like filtering, sorting, and grouping, LINQ in C# is a potent tool for simplifying data searches and manipulations. LINQ may improve code readability, streamline intricate processes, increase efficiency, and lower the probability of mistakes in your projects.